JavaでのintとIntegerの変換:わかりやすく解説
JavaでのintとIntegerの変換の必要性
Javaでは、プリミティブデータ型であるintと、そのラッパークラスであるIntegerが存在します。
これらの間での変換が頻繁に行われるのはなぜでしょうか。今回の記事では、その変換方法と使用例をわかりやすく解説していきます。
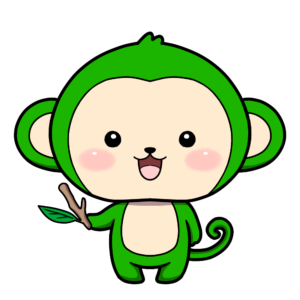
KindleUnlimited会員であれば、全ての本をご覧頂けます。 StreamAPIを理解すれば、Javaの世界が変わる 第1版
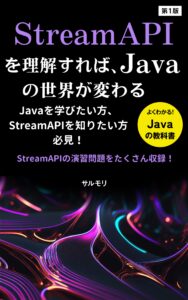
intからIntegerへの変換
intをIntegerに変換するには、Javaのオートボクシング機能を利用するか、Integer.valueOf()
メソッドを使用します。
ソースコード例
1 2 3 4 5 6 7 |
public class Program { public static void main(String[] args) { int primitiveInt = 100; Integer integerObject = Integer.valueOf(primitiveInt); System.out.println(integerObject); } } |
出力結果
100
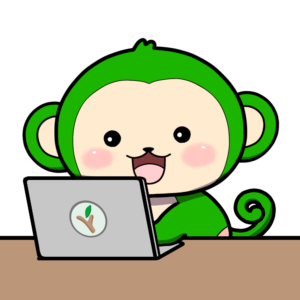
Integerからintへの変換
Integerをintに変換するには、アンボクシングを利用するか、intValue()
メソッドを使用します。
こちらの例を見てみましょう。
ソースコード例
1 2 3 4 5 6 7 |
public class Program { public static void main(String[] args) { Integer integerObject = 200; int primitiveInt = integerObject.intValue(); System.out.println(primitiveInt); } } |
出力結果
200
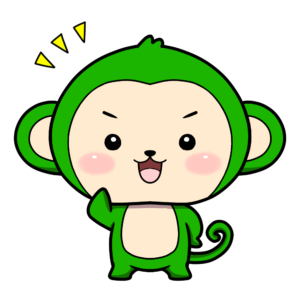
変換時の注意点
intとIntegerの変換時には、特にIntegerがnullの場合に注意が必要です。
nullのIntegerを変換すると、NullPointerExceptionが発生します。
ポイント
変換時の最も一般的なトラップは、NullPointerExceptionです。常に変換前のオブジェクトがnullでないことを確認してから変換を行うようにしましょう。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 |
public class Program { public static void main(String[] args) { Integer integerObject = null; try { int primitiveInt = integerObject.intValue(); System.out.println(primitiveInt); } catch (NullPointerException e) { System.out.println("NullPointer例外が発生しました"); } } } |
出力結果
NullPointer例外が発生しました
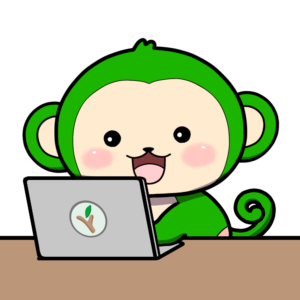
「オートボクシング」と「アンボクシング」の変換
Javaでは、「オートボクシング」と「アンボクシング」という機能を利用して、intとIntegerを相互に変換することができます。
以下に具体的な変換方法を示します。
それぞれ変数に代入ができるんですね。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
public class Program { public static void main(String[] args) { // intからIntegerへの変換(オートボクシング) int i = 10; Integer integerObj = i; // Integerからintへの変換(アンボクシング) Integer anotherIntegerObj = 20; int j = anotherIntegerObj; System.out.println("int i: " + i); System.out.println("Integer integerObj: " + integerObj); System.out.println("Integer anotherIntegerObj: " + anotherIntegerObj); System.out.println("int j: " + j); } } |
出力結果
int i: 10
Integer integerObj: 10
Integer anotherIntegerObj: 20
int j: 20
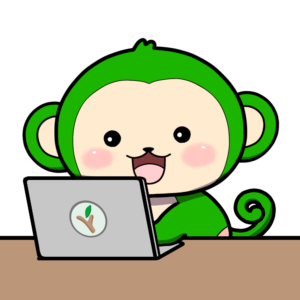
Integerとintの比較方法
int同士の比較は通常の==を使いますが、Integer同士の比較では注意が必要です。
Integer型では==ではなく、equalsメソッドを使用します。
具体的な比較方法を以下に示します。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 |
public class Program { public static void main(String[] args) { int i1 = 100; int i2 = 100; Integer integerObj1 = 100; Integer integerObj2 = 100; System.out.println("i1 == i2: " + (i1 == i2)); System.out.println("integerObj1.equals(integerObj2): " + integerObj1.equals(integerObj2)); } } |
出力結果
i1 == i2: true
integerObj1.equals(integerObj2): true
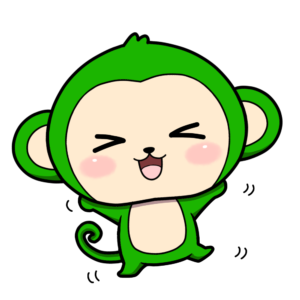
文字列からInteger型に変換するInteger.parseIntメソッド
Javaプログラミングにおいて、文字列から整数への変換が必要になる場合がしばしばあります。ここで、Integer.parseInt
メソッドが非常に便利です。
Integer.parseIntの使用方法
1 2 3 4 5 6 7 |
public class ParseIntExample { public static void main(String[] args) { String numberStr = "42"; int number = Integer.parseInt(numberStr); System.out.println("Parsed integer: " + number); } } |
出力結果
Parsed integer: 42
Integer.parseInt
メソッドは、指定された文字列を引数として受け取り、対応するint値を返します。このメソッドが非常に役立つ場面は、ユーザー入力やファイルから読み取ったデータを数値として扱いたい時などです。
重要な点として、このメソッドは文字列が有効な整数を表していない場合にNumberFormatException
をスローします。したがって、不正な文字列が渡される可能性がある場合は、適切なエラー処理を含めることが良いプラクティスとなります。
-
-
NumberFormatExceptionについてはコチラ【java】NumberFormatExceptionエラーの5つの発生原因と対処方法
「JavaのNumberFormatExceptionを徹底解説!エラー原因と対処法」 はじめに Javaでプログラミングをしていると、数値変換の際に「NumberFormatException」に遭 ...
続きを見る
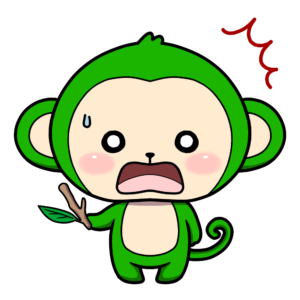
まとめ
JavaにおけるintとIntegerの変換は、日常のコーディングにおいて非常に一般的です。
この記事を通じて、その変換方法や注意点についての理解が深まったことを願っています。
変換時のエラーを避けるためにも、常にnullチェックを行うなど、注意深くコーディングを行うことが大切です。
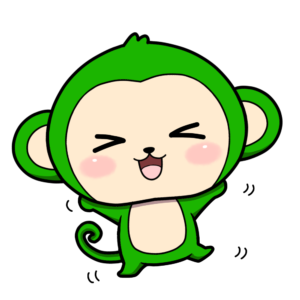
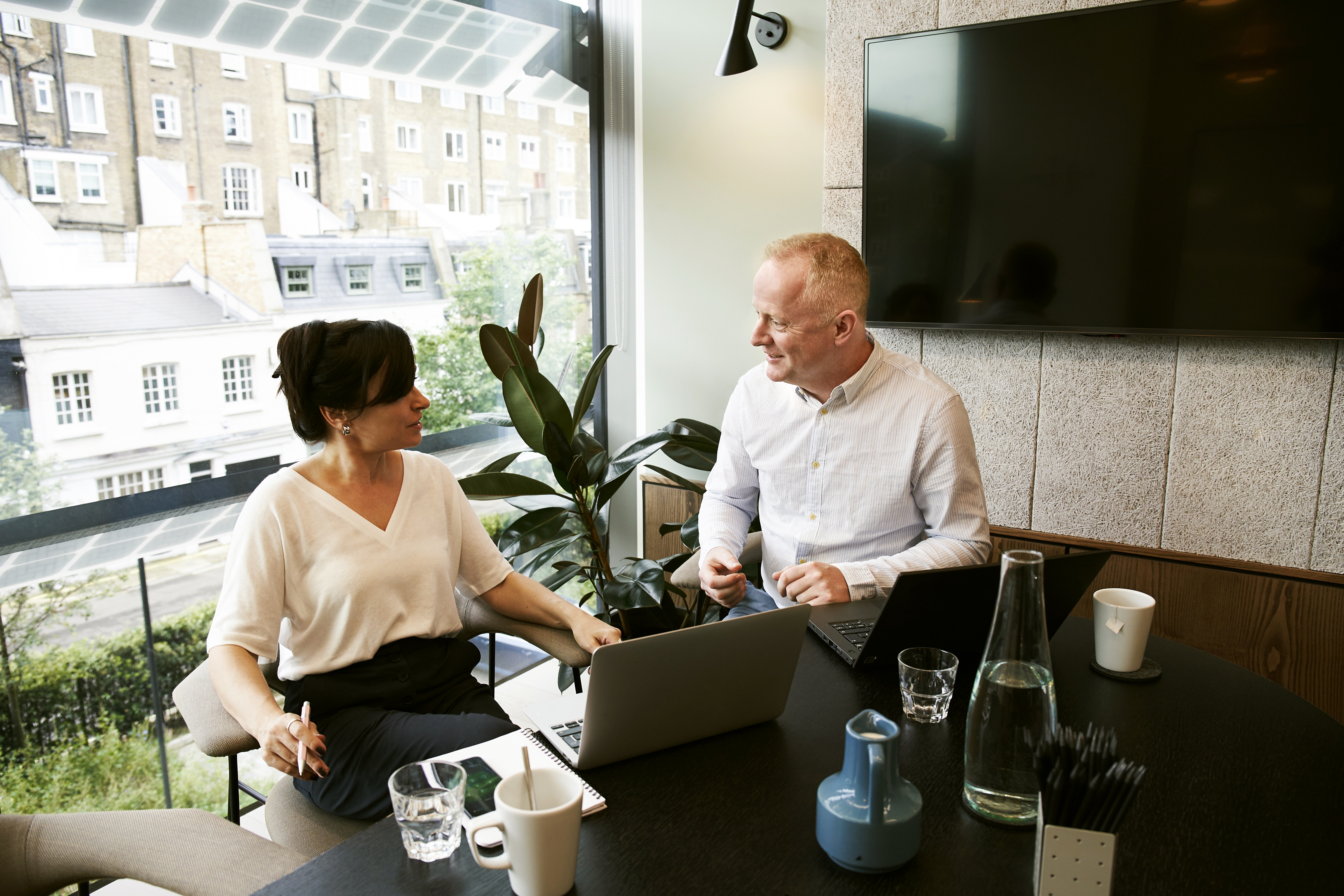
最後まで読んで頂き、ありがとうございました。少しでもお役にたてたなら幸いです!
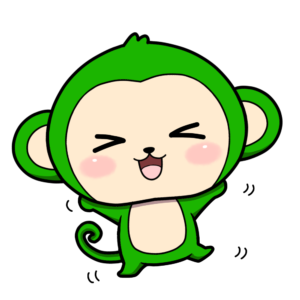