練習問題を始める前に
こんにちは、サルモリです。Linqの練習問題を載せていきます。今回の記事は中級編2です。
イメージがしにくいGroupByの問題を沢山解いてマスターしていきましょう!!
下記に演習問題をまとめてあります。他の問題もどんどん解いてみてください。
他の演習問題はこちら
LinqのGroupByは比較的理解しにくいと思いますが、頑張って知識を付けていってください!
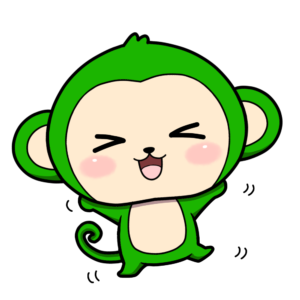
サルモリ
どんどんGroupByの問題を解いていこう!
演習問題を解く前に下記のソースコードをコピーして貼り付け
下記のソースコードを貼り付けてください。Program.csファイルでもRobot.csファイルを作成して貼り付けても問題ありません。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
//下記のロボットクラスをコピーして、貼り付けて下さい。 class Robot { string name, maker, color; int strength; public Robot(string _name,string _maker,string _color,int _strength) { name = _name; maker = _maker; color = _color; strength = _strength; } public string getName() { return name; } public string getMaker() { return maker; } public string getColor() { return color; } public int getstrength() { return strength; } } |
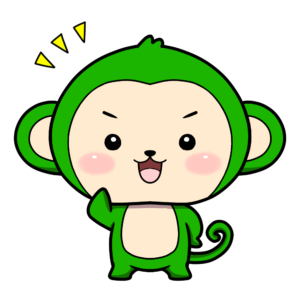
サルモリ
貼り付けたら、演習問題を開始するよ!
第1問
ロボット名をメーカー別に出力してください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
using System; using System.Collections.Generic; using System.Linq; class Program { static void Main(string[] args) { List<Robot> robotlist = new List<Robot>(); robotlist.Add(new Robot("Rockman", "Mr Light", "Blue", 5)); robotlist.Add(new Robot("Quickman", "Mr Wily", "Red", 5)); robotlist.Add(new Robot("Airman", "Mr Wily", "Blue", 3)); robotlist.Add(new Robot("Rollchan", "Mr Light", "Pink", 1)); robotlist.Add(new Robot("Woodman", "Mr Wily", "Green", 3)); robotlist.Add(new Robot("Bubbleman", "Mr Wily", "Green", 4)); robotlist.Add(new Robot("Fireman", "Mr Wily", "Red", 3)); robotlist.Add(new Robot("Iceman", "Mr Wily", "Blue", 4)); robotlist.Add(new Robot("Heatman", "Mr Wily", "Red", 2)); //**************ここからコードを書いて下さい************** var makerrobots = //**************ここまでコードを書いて下さい************** foreach (var robots in makerrobots) { foreach(var robot in robots) { Console.WriteLine(robot.getName()); } Console.WriteLine("-------------"); } } } |
出力結果
Rockman
Rollchan
-------------
Quickman
Airman
Woodman
Bubbleman
Fireman
Iceman
Heatman
-------------
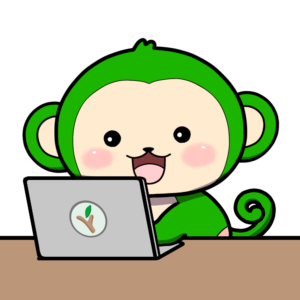
サルモリ
GroupByメソッドの基本だよ!!
第2問
ロボット名を色別に出力してください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
using System; using System.Collections.Generic; using System.Linq; class Program { static void Main(string[] args) { List<Robot> robotlist = new List<Robot>(); robotlist.Add(new Robot("Rockman", "Mr Light", "Blue", 5)); robotlist.Add(new Robot("Quickman", "Mr Wily", "Red", 5)); robotlist.Add(new Robot("Airman", "Mr Wily", "Blue", 3)); robotlist.Add(new Robot("Rollchan", "Mr Light", "Pink", 1)); robotlist.Add(new Robot("Woodman", "Mr Wily", "Green", 3)); robotlist.Add(new Robot("Bubbleman", "Mr Wily", "Green", 4)); robotlist.Add(new Robot("Fireman", "Mr Wily", "Red", 3)); robotlist.Add(new Robot("Iceman", "Mr Wily", "Blue", 4)); robotlist.Add(new Robot("Heatman", "Mr Wily", "Red", 2)); //**************ここからコードを書いて下さい************** var colorrobots = //**************ここまでコードを書いて下さい************** foreach (var robots in colorrobots) { foreach(var robot in robots) { Console.WriteLine(robot.getName() + ":" + robot.getColor()); } Console.WriteLine("-------------"); } } } |
出力結果
Rockman:Blue
Airman:Blue
Iceman:Blue
-------------
Quickman:Red
Fireman:Red
Heatman:Red
-------------
Rollchan:Pink
-------------
Woodman:Green
Bubbleman:Green
-------------