C#リスト操作マスターへの道!IndexOfからBinarySearchまで詳細解説
プログラミング言語C#において、リストは非常に多様で便利な機能を提供しています。
今回は、C#のリスト操作に関する複数のメソッドを詳しく探っていきます。具体的には「IndexOf」、「ToArray」、「TrimExcess」、「Reverse」、「AsReadOnly」、「BinarySearch」に焦点を当てて、それぞれのメソッドの使い方やポイントについて詳しく解説していきます。
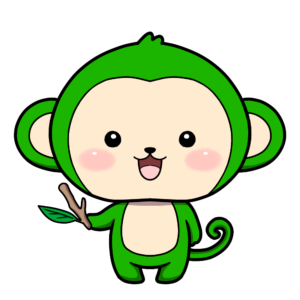
リストの基本メソッドはコチラ
-
-
【C# sharp】リストを徹底解説! 要素の追加、取得、削除、ループを使用した要素の取得、検索方法
ChatGPTのAPIを使わずに自動化することが可能です。 下記の本を読めば、ChatGPT4でも料金掛からずに自動化できます!KindleUnlimited会員であれば無料で読めます。 1. リスト ...
続きを見る
1. 要素の位置を探す!IndexOfメソッド
IndexOfメソッドは、指定された要素がリスト内で最初に出現する位置(インデックス)を返します。
もし、指定した要素がリスト内に存在しない場合は、-1を返します。これを利用して、リスト内に特定の要素が含まれているかを簡単に確認することができます。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 |
using System; using System.Collections.Generic; class Program { static void Main() { List<string> fruits = new List<string>() { "Apple", "Banana", "Cherry", "Date", "Fig" }; int index = fruits.IndexOf("Cherry"); Console.WriteLine("Index of Cherry: " + index); } } |
出力結果
Index of Cherry: 2
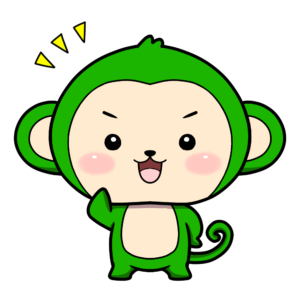
KindleUnlimited会員であれば、全ての本をご覧頂けます。 Linqを理解すれば、C#プログラミングの世界が変わる 第1版
-188x300.jpg)
2. 配列への変換!ToArrayメソッド
ToArrayメソッドを使用すると、リストの要素を配列に変換することができます。
これは、配列とリストを相互に変換し、それぞれのデータ構造のメリットを活かすシチュエーションで非常に役立ちます。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 |
using System; using System.Collections.Generic; class Program { static void Main() { List<string> fruits = new List<string>() { "Apple", "Banana", "Cherry", "Date", "Fig" }; string[] fruitArray = fruits.ToArray(); Console.WriteLine("Fruit Array: " + string.Join(", ", fruitArray)); } } |
出力結果
Fruit Array: Apple, Banana, Cherry, Date, Fig
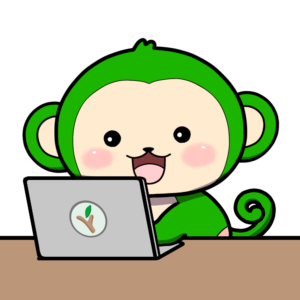
3. リストの容量を調整する!TrimExcessメソッド
TrimExcessメソッドは、リストの容量を実際の要素数に適したサイズに切り詰めるメソッドです。
リストの容量は、要素が追加されるときに自動で増加しますが、要素が削除されたときには自動で減少しません。TrimExcessを使用して、使用されていないメモリを解放することができます。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
using System; using System.Collections.Generic; class Program { static void Main() { List<int> numbers = new List<int>(100); numbers.Add(1); numbers.Add(2); numbers.Add(3); Console.WriteLine("Capacity before TrimExcess: " + numbers.Capacity); numbers.TrimExcess(); Console.WriteLine("Capacity after TrimExcess: " + numbers.Capacity); } } |
出力結果
Capacity before TrimExcess: 100 Capacity after TrimExcess: 3
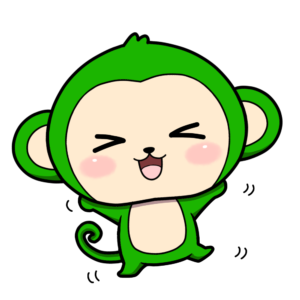
4. リストの要素を反転!Reverseメソッド
Reverseメソッドは、リスト内の要素の順序を反転させます。
これにより、最初の要素が最後に、最後の要素が最初に移動します。このメソッドは、要素を逆順に処理したい場合に便利です。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
using System; using System.Collections.Generic; class Program { static void Main() { List<string> fruits = new List<string>() { "Apple", "Banana", "Cherry", "Date", "Fig" }; Console.WriteLine("Before Reverse: " + string.Join(", ", fruits)); fruits.Reverse(); Console.WriteLine("After Reverse: " + string.Join(", ", fruits)); } } |
出力結果
Before Reverse: Apple, Banana, Cherry, Date, Fig After Reverse: Fig, Date, Cherry, Banana, Apple
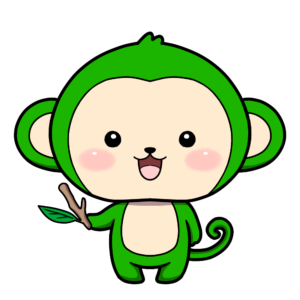
5. リストを読み取り専用に!AsReadOnlyメソッド
AsReadOnlyメソッドを使用すると、リストを読み取り専用に変換できます。
読み取り専用のコレクションを作成することで、そのコレクションが変更できないようにし、データの安全性を確保することができます。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 |
using System; using System.Collections.Generic; using System.Collections.ObjectModel; class Program { static void Main() { List<string> fruits = new List<string>() { "Apple", "Banana", "Cherry" }; ReadOnlyCollection<string> readOnlyFruits = fruits.AsReadOnly(); Console.WriteLine("Read-only list: " + string.Join(", ", readOnlyFruits)); } } |
出力結果
Read-only list: Apple, Banana, Cherry
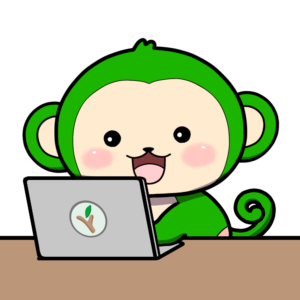
6. 二分探索法で要素を検索!BinarySearchメソッド
BinarySearchメソッドを使用して、ソートされたリスト内で指定した要素を二分探索法を使用して検索することができます。
このメソッドは、リストがすでにソートされている場合に非常に効率的な検索が可能です。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
using System; using System.Collections.Generic; class Program { static void Main() { List<int> numbers = new List<int>() { 1, 3, 5, 7, 9 }; int targetNumber = 5; int index = numbers.BinarySearch(targetNumber); if (index >= 0) { Console.WriteLine($"{targetNumber} was found at index {index}."); } else { Console.WriteLine($"{targetNumber} was not found."); } } } |
出力結果
5 was found at index 2.
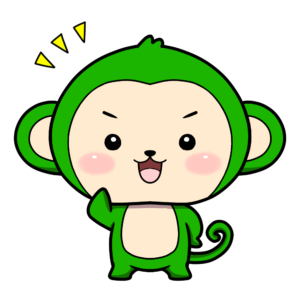
7. まとめ
以上、C#のListクラスでよく使用されるメソッド、IndexOf、ToArray、TrimExcess、Reverse、AsReadOnly、BinarySearchについて見てきました。
これらのメソッドは、リストを操作する際の基本となりますので、ぜひ実際にコードを書いて実行してみて、機能を把握してくださいね!
最後まで読んで頂き、ありがとうございました。少しでもお役にたてたなら幸いです!
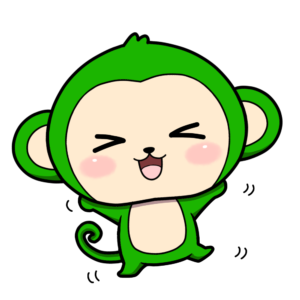