Dictionary型について
今回のテーマ
こんばんは、サルモリです。
今回は下記のテーマについての記事を書いていこうと思います。
今回のテーマ
Dictionaryの使い方について解説。
今回はディクショナリーの使い方を解説します。リストや配列程、使う機会はないかもしれませんが、便利なので覚えていきましょう!
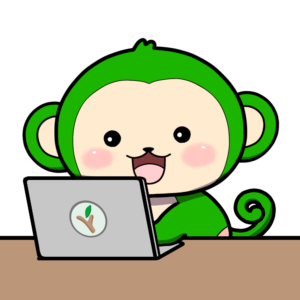
Dictionaryの初期化方法
まずはDictionaryの初期化方法を見ていきましょう。2パターンあるので、好きな方を選んでください。
今回の例では、ロボット名がキーで、それに対応する要素名が作成者とします。
初期化方法1
1 2 3 4 5 6 |
var RobotDict = new Dictionary<string, string>(){ {"Rockman","Dr Light" }, {"Roll","Dr Light" }, {"Airman","Dr Wily" }, {"Quickman","Dr Wily" } }; |
初期化方法2
1 2 3 4 5 6 |
var RobotDict = new Dictionary<string, string>(){ ["Rockman"] = "Dr Light", ["Roll"] = "Dr Light", ["Airman"] = "Dr Wily", ["Quickman"] = "Dr Wily" }; |
このブログでは、初期化方法2を使っていきますね。
Dictionaryの要素の追加方法
要素の追加方法も2つあります。
要素の追加方法1
1 |
RobotDict["Iceman"] = "Dr Wily"; |
要素の追加方法2
1 |
RobotDict.Add("Iceman", "Dr Wily"); |
要素の追加方法1では、既にキーが存在していた場合は、値が上書きされます。
追加方法2では、既にキーが存在していた場合、ArgumentException例外が発生するので注意が必要です。
このブログでは、追加方法1を使っていきます。
Dictionaryから要素を取り出す方法
続けて、要素の取り出し方です。下記のようにすれば、キーに対応した値を取り出すことができます。
1 |
var maker = RobotDict["Rockman"]; |
指定したキーが無かった場合はKeyNotFoundException例外が発生するので、ContainsKeyメソッドを使うことで、キーが存在するかを確認することをおすすめします。
1 2 3 4 |
if (RobotDict.ContainsKey("Rockman")) { var maker = RobotDict["Rockman"]; } |
Dictionaryから要素を削除する方法
要素の削除方法を解説します。下記のようにRemoveメソッドを使用することで削除できます。
1 |
var result = RobotDict.Remove("Rockman"); |
返り値はbool型で削除出来た場合はtrue、出来なかった場合はfalseを返します。
Dictionaryから全ての要素を取り出す方法
全ての要素を取り出す場合はforeachを使いましょう。キーの値はKeyを指定し、それに対応する要素の値はValueを指定します。
今までの処理を総まとめしたソースコードを記述します。
Dictionaryの使い方
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
using System; using System.Collections.Generic; namespace Dictionary { class Program { static void Main(string[] args) { var RobotDict = new Dictionary<string, string>() { ["Rockman"] = "Dr Light", ["Roll"] = "Dr Light", ["Airman"] = "Dr Wily", ["Quickman"] = "Dr Wily" }; RobotDict["Iceman"] = "Dr Wily"; var maker = RobotDict["Rockman"]; Console.WriteLine(maker); RobotDict.Remove("Rockman"); foreach(var item in RobotDict) { Console.WriteLine(item.Key + ":" + item.Value); } } } } |
result
Dr Light
Roll:Dr Light
Airman:Dr Wily
Quickman:Dr Wily
Iceman:Dr Wily
全ての要素を取り出す時にforeachを使えるということは、Linqも使用することができます。
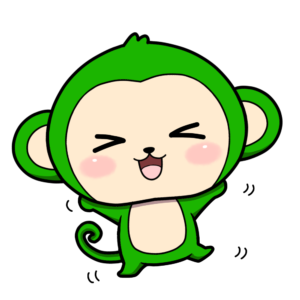
KindleUnlimited会員であれば、全ての本をご覧頂けます。 Linqを理解すれば、C#プログラミングの世界が変わる 第1版
-188x300.jpg)
Dictionary型にLinqを使用する
Dictionary型にLinqを使用した例
最後にLinqを使ってみた例を二つ紹介します。
行いたい処理
文字列の長さが7以上のロボット名と作成者を出力する。
Linqを使用する例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
using System; using System.Collections.Generic; using System.Linq; namespace Dictionary { class Program { static void Main(string[] args) { var RobotDict = new Dictionary<string, string>() { ["Rockman"] = "Dr Light", ["Roll"] = "Dr Light", ["Airman"] = "Dr Wily", ["Quickman"] = "Dr Wily", ["Iceman"] = "Dr Wily", ["Fireman"] = "Dr Wily" }; RobotDict = RobotDict.Where(x => x.Key.Length >= 7).ToDictionary(robot => robot.Key,robot => robot.Value); foreach(var item in RobotDict) { Console.WriteLine(item.Key + ":" + item.Value); } } } } |
result
Rockman:Dr Light
Quickman:Dr Wily
Fireman:Dr Wily
Linqを使用して、Whereで抽出した後にToDirectoryで再度、Directory型に戻しています。
上記のようにToDirectory内でそれぞれKeyとValueを指定することでDirectory型を再度作ることができます。
LinqのWhereについては下記の記事に書いています。
CHECK
-
-
【C# sharp Linq】Selectメソッド、Whereメソッドを解説します。
ChatGPTのAPIを使わずに自動化することが可能です。 下記の本を読めば、ChatGPT4でも料金掛からずに自動化できます!KindleUnlimited会員であれば無料で読めます。 C#言語のL ...
続きを見る
二つ目の例を見てみましょう。
行いたい処理
作成者がワイリーのロボット名を出力する。
Linqを使用する例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
using System; using System.Collections.Generic; using System.Linq; namespace Dictionary { class Program { static void Main(string[] args) { var RobotDict = new Dictionary<string, string>() { ["Rockman"] = "Dr Light", ["Roll"] = "Dr Light", ["Airman"] = "Dr Wily", ["Quickman"] = "Dr Wily", ["Iceman"] = "Dr Wily", ["Fireman"] = "Dr Wily" }; var RobotList = RobotDict.Where(x => x.Value == "Dr Wily").Select(x => x.Key).ToList(); foreach(var item in RobotList) { Console.WriteLine(item); } } } } |
result
Airman
Quickman
Iceman
Fireman
今回の例はロボット名の出力のみだったので、リストに変換して実現しました。
もちろん、Dictionary型に変換し、出力することもできます。時と場合に応じて、使い分けましょう。
Dictionary型にクラスを使用する
これからはクラスを定義し、クラスを格納したDictionary型の使い方をみていきましょう!
使い方を紹介する上でクラスの定義が必要なので、今回は下記のクラスを使用します。何回か使用したことがあるロボットクラスです。
使用するクラスの定義
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
class Robot { string name, color, maker; int strength; public Robot(string _name, string _color, int _strength, string _maker) { name = _name; color = _color; strength = _strength; maker = _maker; } public String Name { get { return name; } } public String Color { get { return color; } } public int Strength { get { return strength; } } public String Maker { get { return maker; } } } |
Dictionaryに定義したクラスのオブジェクトを格納する
早速、上記のクラスをDictionaryに格納してみましょう。
1 2 3 4 5 6 7 8 9 |
var RobotDict = new Dictionary<string, Robot>() { ["0001"] = new Robot("Rockman","Blue",5, "Dr Light"), ["0002"] = new Robot("Roll", "Pink", 1, "Dr Light"), ["0003"] = new Robot("Airman", "Blue", 3, "Dr Wily"), ["0004"] = new Robot("Quickman", "Red", 5, "Dr Wily"), ["0005"] = new Robot("Iceman", "Blue", 4, "Dr Wily"), ["0006"] = new Robot("FIreman", "Red", 4, "Dr Wily"), }; |
今回はKeyをロボットコードとして、要素をロボットオブジェクトとして生成し、格納してみました。
Dictionaryからクラスで定義したオブジェクトを取り出す方法
クラスで定義したオブジェクトを取り出してみましょう。
クラスで定義したロボット名を出力
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
using System; using System.Collections.Generic; using System.Linq; namespace Dictionary { class Program { static void Main(string[] args) { var RobotDict = new Dictionary<string, Robot>() { ["0001"] = new Robot("Rockman","Blue",5, "Dr Light"), ["0002"] = new Robot("Roll", "Pink", 1, "Dr Light"), ["0003"] = new Robot("Airman", "Blue", 3, "Dr Wily"), ["0004"] = new Robot("Quickman", "Red", 5, "Dr Wily"), ["0005"] = new Robot("Iceman", "Blue", 4, "Dr Wily"), ["0006"] = new Robot("FIreman", "Red", 4, "Dr Wily"), }; foreach(var robot in RobotDict) { Console.WriteLine(robot.Key + ":" + robot.Value.Name); } } } } |
result
0001:Rockman
0002:Roll
0003:Airman
0004:Quickman
0005:Iceman
0006:FIreman
今回の例では、キーの値と要素の中のロボット名を出力しています。
DictionaryでLinqを使用した例 OrderbyとGroupby
クラスで定義したオブジェクトに対して、Linqを使用してみましょう。
やりたい処理
強さ順に並べ替えたDictionaryを作成したい。
クラスで定義したロボット名を出力
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
using System; using System.Collections.Generic; using System.Linq; namespace Dictionary { class Program { static void Main(string[] args) { var RobotDict = new Dictionary<string, Robot>() { ["0001"] = new Robot("Rockman","Blue",5, "Dr Light"), ["0002"] = new Robot("Roll", "Pink", 1, "Dr Light"), ["0003"] = new Robot("Airman", "Blue", 3, "Dr Wily"), ["0004"] = new Robot("Quickman", "Red", 5, "Dr Wily"), ["0005"] = new Robot("Iceman", "Blue", 4, "Dr Wily"), ["0006"] = new Robot("FIreman", "Red", 4, "Dr Wily"), }; RobotDict = RobotDict.OrderByDescending(x => x.Value.Strength).ToDictionary(robot => robot.Key, robot => robot.Value); foreach(var robot in RobotDict) { Console.WriteLine(robot.Key + ":" + robot.Value.Name + ":" + robot.Value.Strength); } } } } |
result
0001:Rockman:5
0004:Quickman:5
0005:Iceman:4
0006:FIreman:4
0003:Airman:3
0002:Roll:1
強さ順に並べ替えができました。
もう一つ、色でグループ分けしたDictionaryを作成してみましょう。
やりたい処理
色でグループ分けしたDictionaryを作成したい。
クラスで定義したロボット名を出力
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
using System; using System.Collections.Generic; using System.Linq; namespace Dictionary { class Program { static void Main(string[] args) { var RobotDict = new Dictionary<string, Robot>() { ["0001"] = new Robot("Rockman","Blue",5, "Dr Light"), ["0002"] = new Robot("Roll", "Pink", 1, "Dr Light"), ["0003"] = new Robot("Airman", "Blue", 3, "Dr Wily"), ["0004"] = new Robot("Quickman", "Red", 5, "Dr Wily"), ["0005"] = new Robot("Iceman", "Blue", 4, "Dr Wily"), ["0006"] = new Robot("FIreman", "Red", 4, "Dr Wily"), }; var RobotDictList = RobotDict.GroupBy(x => x.Value.Color); foreach(var robotdict in RobotDictList) { foreach(var robot in robotdict) { Console.WriteLine(robot.Key + ":" + robot.Value.Name + ":" + robot.Value.Color); } Console.WriteLine("------------------------"); } } } } |
result
0001:Rockman:Blue
0003:Airman:Blue
0005:Iceman:Blue
------------------------
0002:Roll:Pink
------------------------
0004:Quickman:Red
0006:FIreman:Red
------------------------
色でグループ分けをすることができましたね。
配列、リストなどの記事はこちら
Linqを使用してリストからDictionary型への変換
最後にリストからDictionaryへの変換について解説します。
まず、下記のようにロボットのリストを作ってみました。
ロボットリスト
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
using System; using System.Collections.Generic; using System.Linq; namespace Dictionary { class Program { static void Main(string[] args) { var robotlist = new List<Robot>(); robotlist.Add(new Robot("Rockman", "Blue", 5, "Dr Light")); robotlist.Add(new Robot("Roll", "Pink", 1, "Dr Light")); robotlist.Add(new Robot("Airman", "Blue", 3, "Dr Wily")); robotlist.Add(new Robot("Quickman", "Red", 5, "Dr Wily")); robotlist.Add(new Robot("Iceman", "Blue", 4, "Dr Wily")); robotlist.Add(new Robot("Fireman", "Red", 4, "Dr Wily")); foreach(var robot in robotlist){ Console.WriteLine(robot.Name); } } } } |
result
Rockman
Roll
Airman
Quickman
Iceman
Fireman
上記のリストをLinqを使用してDictionary型に変換してみます。
ロボットリストをロボットDictionaryに変換
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
using System; using System.Collections.Generic; using System.Linq; namespace Dictionary { class Program { static void Main(string[] args) { var robotlist = new List<Robot>(); robotlist.Add(new Robot("Rockman", "Blue", 5, "Dr Light")); robotlist.Add(new Robot("Roll", "Pink", 1, "Dr Light")); robotlist.Add(new Robot("Airman", "Blue", 3, "Dr Wily")); robotlist.Add(new Robot("Quickman", "Red", 5, "Dr Wily")); robotlist.Add(new Robot("Iceman", "Blue", 4, "Dr Wily")); robotlist.Add(new Robot("Fireman", "Red", 4, "Dr Wily")); var robotDict = robotlist.ToDictionary(robot => robot.Name); Console.WriteLine("Icemancolor = " + robotDict["Iceman"].Color); Console.WriteLine("-------------------------------"); foreach(var robot in robotDict){ Console.WriteLine(robot.Key + ":" + robot.Value.Color + ":" + robot.Value.Maker); } } } } |
result
Icemancolor = Blue
-------------------------------
Rockman:Blue:Dr Light
Roll:Pink:Dr Light
Airman:Blue:Dr Wily
Quickman:Red:Dr Wily
Iceman:Blue:Dr Wily
Fireman:Red:Dr Wily
上記のToDirectory内で何をしているか解説すると、キーをロボット名で定義しています。
なので、Directoryのキーをロボット名で指定すると対応した値を取り出すことが可能です。
下記の疑問についてもお答えします。
そもそもリストをDictionaryにする必要あるの?
仕事でプログラミングをしていた時、使う場面は結構あります。どの場面で使ったかというと、性能改善の時です。
Dictionary型にすると、キーを指定することで一発で要素の取得が出来るのでその特性を活かして、性能改善をすることができます。
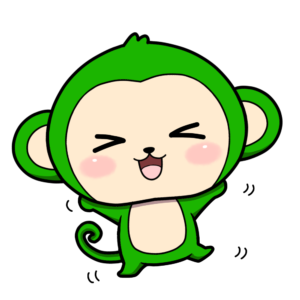
まとめ
今回もDictionary型について解説しました。最後のサルモリが言ってることがとても重要です。
開発では、配列、リストをメインに使うことが多いと思いますが、その時の課題に応じて、Dictionaryを使用することも視野にいれましょう。
今回の2記事でDictionaryを使いこなすことが出来ると思うので、何回も見直してぜひ覚えてください。
最後まで見て頂きありがとうございました。
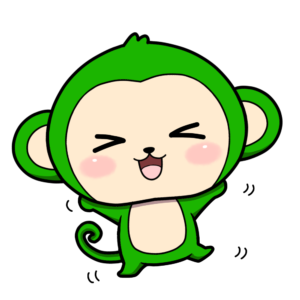
今回はDictionary型について解説しました。
二つの要素を対応付けて管理したい場合などにとても強力ないないので、ぜひ覚えてください。
今回の記事では、Dictionary型について紹介しきれていないので、別記事でさらに掘り下げて解説していきたいと思います。
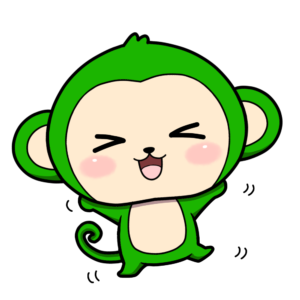
他のLinqの記事についてはこちら