今回のテーマ
今回の記事では、List<T>クラスのラムダ式を引数に持っているメソッドをピックアップしてみます。
ピックアップするのは下記のメソッドです。
ラムダ式と組み合わせるメソッド一覧
- Exists
- Find
- FindAll
- FindIndex
- FindLast
- FindLastIndex
数が多いですが、頑張って使用例を作っていきたいと思います。ぜひ、参考にしてくださいね。
ラムダ式については下記に詳しく書いていますので、良ければみてください。
CHECK
-
-
【C# sharp】匿名メソッド、ラムダ式(=>)について解説します。
ChatGPTのAPIを使わずに自動化することが可能です。 下記の本を読めば、ChatGPT4でも料金掛からずに自動化できます!KindleUnlimited会員であれば無料で読めます。 まえがき こ ...
続きを見る
Exists
まずはExistsです。メタデータで覗いてみると、下記のようなメソッドでした。
1 |
public bool Exists(Predicate<T> match); |
引数にラムダ式を指定して、一致している場合は、bool型で返してくれるみたいですね。
早速参考となるソースコードを書いてみます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { List<string> robotlist = new List<string>(); robotlist.Add("Rockman"); robotlist.Add("Rollchan"); robotlist.Add("Fireman"); robotlist.Add("Iceman"); robotlist.Add("Airman"); Console.WriteLine(robotlist.Exists(n => n == "Rockman")); } } |
出力結果
True
リスト内の要素を調べて、一致してたらTrueを返してくれます。
下記のようにStartWithメソッドなどと組み合わせて使うのも良さそうですね。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { List<string> robotlist = new List<string>(); robotlist.Add("Rockman"); robotlist.Add("Rollchan"); robotlist.Add("Fireman"); robotlist.Add("Iceman"); robotlist.Add("Airman"); Console.WriteLine(robotlist.Exists(n => n.StartsWith("F"))); } } |
出力結果
True
Find
Findメソッドは最初に引数に指定した要素を返すメソッドです。下記にようなメソッドとなっています。
1 |
public T Find(Predicate<T> match); |
引数にラムダ式を指定し、要素と一致していたらその要素を返してくれるみたいですね。
早速例として下記のソースコードの動きを確認してみます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { List<string> robotlist = new List<string>(); robotlist.Add("Rockman"); robotlist.Add("Rollchan"); robotlist.Add("Fireman"); robotlist.Add("Iceman"); robotlist.Add("Airman"); Console.WriteLine(robotlist.Find(n => n == "Fireman")); } } |
出力結果
Fireman
一致しているFiremanを返しましたね。今度はStartWithメソッドを使用して下記のように指定してみます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { List<string> robotlist = new List<string>(); robotlist.Add("Rockman"); robotlist.Add("Rollchan"); robotlist.Add("Fireman"); robotlist.Add("Iceman"); robotlist.Add("Airman"); Console.WriteLine(robotlist.Find(n => n.StartsWith("R"))); } } |
出力結果
Rockman
初めに一致した値のみ返すことが分かります。
因みにどの要素にも一致しない場合は、空文字を返しました。
他のLinqの記事についてはこちら
FindAll
続けて、FindAllです。用途はFindメソッドに似てますが、このメソッドは一致している全ての要素を返してくれます。
一応、メソッドを確認してみましょう。
1 |
public List<T> FindAll(Predicate<T> match); |
一致した要素をリストとして返してくれるのが分かりますね。便利です。
Findメソッドで紹介した二つ目のソースコードを少し変更し、下記のようにしてみました。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { List<string> robotlist = new List<string>(); robotlist.Add("Rockman"); robotlist.Add("Rollchan"); robotlist.Add("Fireman"); robotlist.Add("Iceman"); robotlist.Add("Airman"); foreach(var robot in robotlist.FindAll(n => n.StartsWith("R"))) { Console.WriteLine(robot); } } } |
出力結果
Rockman
Rollchan
「R」から始まる全てのロボット名が出力されましたね。
個人的にFindメソッドよりもFindAllメソッドの方が便利だと思います。
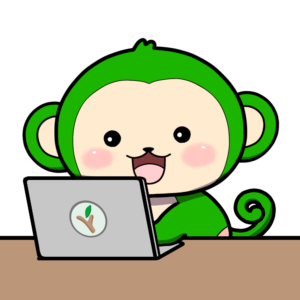
FindIndex
FindIndexメソッドも今まで通りに用途は似ています。
メソッドとしては下記の引数のパターンが用意されています。
1 2 3 |
public int FindIndex(int startIndex, int count, Predicate<T> match); public int FindIndex(int startIndex, Predicate<T> match); public int FindIndex(Predicate<T> match); |
今回は引数がラムダ式の一つのパターンのみしか紹介しませんが、startIndexは検索を始める位置、countは検索する個数を表します。
戻り値はint型なので、要素の値が一致した位置の番号を返すメソッドのようですね。例となるソースコードを書いていきます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { List<string> robotlist = new List<string>(); robotlist.Add("Rockman"); robotlist.Add("Rollchan"); robotlist.Add("Fireman"); robotlist.Add("Iceman"); robotlist.Add("Airman"); Console.WriteLine(robotlist.FindIndex(n => n.StartsWith("F"))); } } |
出力結果
2
一致した「Fireman」の要素番号が返ってきていますね。因みにどの要素にも一致しない場合は、-1が返ります。
FindLast
Findメソッドは前から検索していきますが、FindLastメソッドは後ろから検索をしていきます。
下記のソースコードを見れば、その通りになっていることが分かると思います。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Collections.Generic; class Program { static void Main(string[] args) { List<string> robotlist = new List<string>(); robotlist.Add("Rockman"); robotlist.Add("Rollchan"); robotlist.Add("Fireman"); robotlist.Add("Iceman"); robotlist.Add("Airman"); Console.WriteLine(robotlist.FindLast(n => n.StartsWith("R"))); } } |
出力結果
Rollchan
後ろから検索しているので、「Rollchan」が出力されていますね。Findメソッドと一緒に覚えておきましょう。
FindLastIndex
FindIndexが前から検索するので、FindLastIndexは後ろから検索します。
以下略・・・。
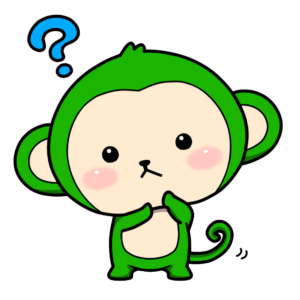
他のLinqの記事についてはこちら
まとめ
今回はラムダ式と組み合わせることが出来る下記のリストのメソッドを紹介しました。
今回紹介したメソッド
- Exists
- Find
- FindAll
- FindIndex
- FindLast
- FindLastIndex
あと3つラムダ式と組み合わせることができるメソッドが3つあるので、別の記事で紹介します。
今回紹介した中でFindAllは特に便利なので、ぜひ覚えてくださいね。
それでは、最後まで読んでくれてありがとうございました。
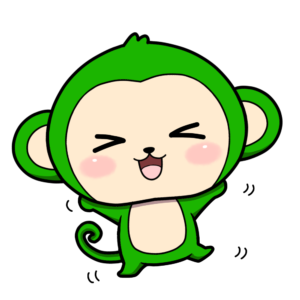
ラムダ式については下記に詳しく書いているので、併せて読んでみてください。
配列、リストなどの記事はこちら
CHECK
-
-
【C# sharp】匿名メソッド、ラムダ式(=>)について解説します。
ChatGPTのAPIを使わずに自動化することが可能です。 下記の本を読めば、ChatGPT4でも料金掛からずに自動化できます!KindleUnlimited会員であれば無料で読めます。 まえがき こ ...
続きを見る