はじめに: C#のString型のIsInternedメソッドとは?
C#のString型のIsInternedメソッドとは何でしょうか?
IsInternedメソッドは、指定した文字列がインターンされているかどうかを調べるためのメソッドです。インターンとは、一度生成した文字列をプールして再利用することで、メモリの効率的な利用を可能にする仕組みのことを言います。
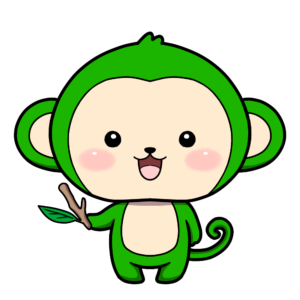
KindleUnlimited会員であれば、全ての本をご覧頂けます。 Linqを理解すれば、C#プログラミングの世界が変わる 第1版
-188x300.jpg)
IsInternedメソッドの基本的な使用方法
それでは早速、IsInternedメソッドの基本的な使用方法について説明しましょう。
IsInternedメソッドは、文字列がインターンされている場合にその文字列を返し、そうでない場合にはnullを返します。以下に簡単な使用例を示します。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
using System; class Program { static void Main() { string str1 = "Hello, world!"; string str2 = string.IsInterned(str1); if (str2 != null) { Console.WriteLine(str2 + " is interned."); } else { Console.WriteLine(str1 + " is not interned."); } } } |
出力結果
Hello, world! is interned.
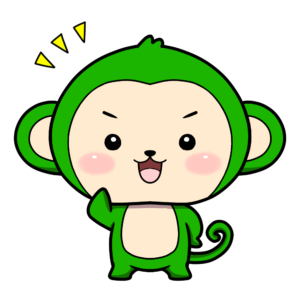
IsInternedメソッドの詳細な使用方法
次に、IsInternedメソッドの詳細な使用方法について見ていきましょう。
例えば、同じ内容の文字列を複数生成した場合、それらは全て同じインスタンスを参照します。これを確認するためにIsInternedメソッドを利用することができます。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
using System; class Program { static void Main() { string str1 = "Hello, world!"; string str2 = "Hello, world!"; string str3 = string.IsInterned(str1); string str4 = string.IsInterned(str2); if (str3 != null && str4 != null) { Console.WriteLine("Both strings are interned."); } else { Console.WriteLine("One or both strings are not interned."); } } } |
出力結果
Both strings are interned.
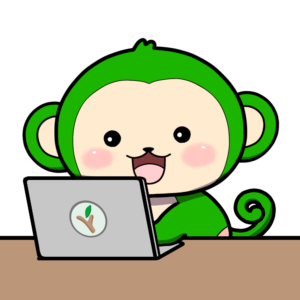
IsInternedメソッドの注意点
しかし、注意しなければならない点が一つあります。
それは、IsInternedメソッドは文字列リテラルに対してのみインターンを保証するということです。文字列操作によって生成された文字列はインターンされない可能性があります。これを確認するためのコードを以下に示します。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
using System; class Program { static void Main() { string str1 = "Hello, world!"; string str2 = "Hello, " + "world!"; string str3 = string.IsInterned(str1); string str4 = string.IsInterned(str2); if (str3 != null && str4 != null) { Console.WriteLine("Both strings are interned."); } else { Console.WriteLine("One or both strings are not interned."); } } } |
出力結果
One or both strings are not interned.
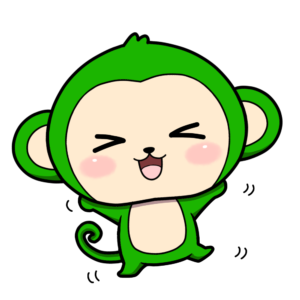
まとめ
以上がC#のString型のIsInternedメソッドの使い方についての説明でした。
IsInternedメソッドは、指定した文字列がインターンされているかどうかを調べるためのメソッドで、文字列がインターンされている場合にその文字列を返し、そうでない場合にはnullを返します。
また、IsInternedメソッドは文字列リテラルに対してのみインターンを保証し、文字列操作によって生成された文字列はインターンされない可能性があるという点を覚えておくと良いでしょう。
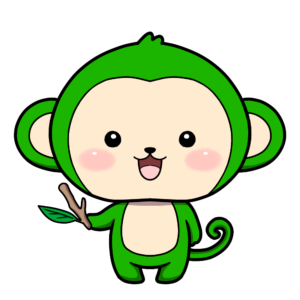
最後まで読んで頂き、ありがとうございました。少しでもお役に立てたなら幸いです!
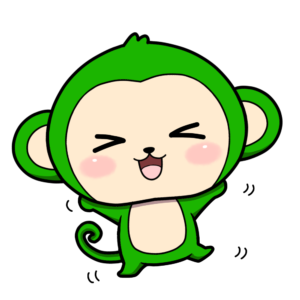