1. 辞書型とは
C#において、辞書型(Dictionary型)はキーと値のペアを保存するためのデータ型です。
これは非常に強力で、項目を素早く検索する能力があります。
各キーは一意でなければならず、一つの辞書内で二度同じキーを使用することはできません。
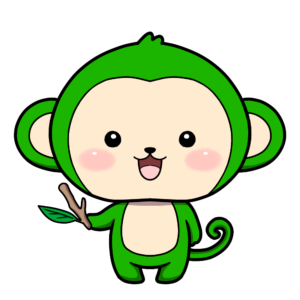
KindleUnlimited会員であれば、全ての本をご覧頂けます。 Linqを理解すれば、C#プログラミングの世界が変わる 第1版
-188x300.jpg)
2. 辞書型の宣言と初期化
辞書型の宣言は以下のように行います。ここでは、キーがstring型、値がint型の辞書を作成します。
ソースコード例
1 2 3 4 5 6 7 8 |
using System; using System.Collections.Generic; class Program { static void Main() { Dictionary<string, int> dictionary = new Dictionary<string, int>(); } } |
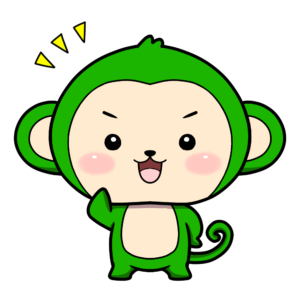
3. 要素の追加
辞書に要素を追加するには、Addメソッドを使用します。
以下の例では、新たにキーと値のペアを追加しています。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 |
using System; using System.Collections.Generic; class Program { static void Main() { Dictionary<string, int> dictionary = new Dictionary<string, int>(); dictionary.Add("apple", 1); dictionary.Add("banana", 2); dictionary.Add("cherry", 3); } } |
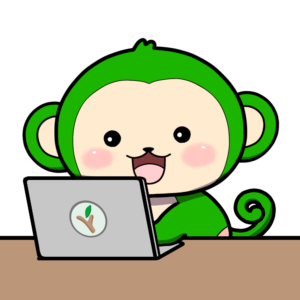
4. 要素の取得
辞書から要素を取得するには、キーを使って直接アクセスします。
以下の例では、キー"banana"に対応する値を取得しています。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
using System; using System.Collections.Generic; class Program { static void Main() { Dictionary<string, int> dictionary = new Dictionary<string, int>(); dictionary.Add("apple", 1); dictionary.Add("banana", 2); dictionary.Add("cherry", 3); Console.WriteLine(dictionary["banana"]); } } |
出力結果
2
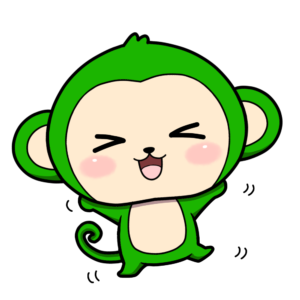
5. 要素の修正
辞書の要素を修正するには、取得と同じくキーを使って直接アクセスします。
以下の例では、キー"banana"に対応する値を5に変更しています。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Collections.Generic; class Program { static void Main() { Dictionary<string, int> dictionary = new Dictionary<string, int>(); dictionary.Add("apple", 1); dictionary.Add("banana", 2); dictionary.Add("cherry", 3); dictionary["banana"] = 5; Console.WriteLine(dictionary["banana"]); } } |
出力結果
5
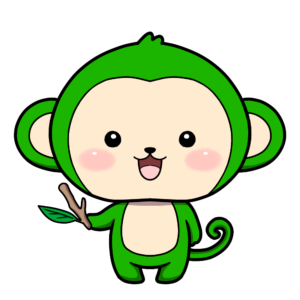
6. 要素の削除
辞書から要素を削除するには、Removeメソッドを使用します。
以下の例では、キー"banana"とその値を辞書から削除しています。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
using System; using System.Collections.Generic; class Program { static void Main() { Dictionary<string, int> dictionary = new Dictionary<string, int>(); dictionary.Add("apple", 1); dictionary.Add("banana", 2); dictionary.Add("cherry", 3); dictionary.Remove("banana"); } } |
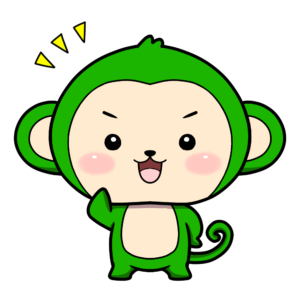
7. ループを使った参照
辞書内のすべての要素をループで参照するには、foreachを使うのが一般的です。
以下の例では、すべてのキーと値を表示しています。
ソースコード例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
using System; using System.Collections.Generic; class Program { static void Main() { Dictionary<string, int> dictionary = new Dictionary<string, int>(); dictionary.Add("apple", 1); dictionary.Add("banana", 2); dictionary.Add("cherry", 3); foreach (KeyValuePair<string, int> kvp in dictionary) { Console.WriteLine("Key = {0}, Value = {1}", kvp.Key, kvp.Value); } } } |
出力結果
Key = apple, Value = 1
Key = banana, Value = 2
Key = cherry, Value = 3
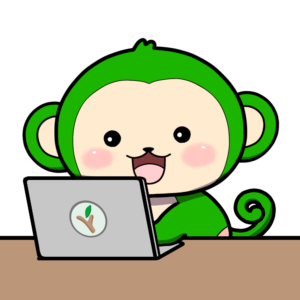
まとめ
C#の辞書型は、キーと値をペアにしてデータを保存するための強力なツールです。
要素の取得、修正、追加、削除、そしてループを使った参照など、さまざまな操作が可能です。
これらの基本的な操作を理解し、自分のコードに適用することで、より効率的なプログラミングが可能になります。
最後まで読んで頂き、ありがとうございました。少しでもお役にたてたなら幸いです!
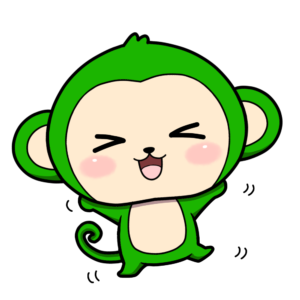